Note
This notebook can be downloaded here: 08_Image_Processing_Practical_Work.ipynb
Practical Work: Steel microstructure¶
In this tutorial, you will study the microstructure of an HSLA steel.
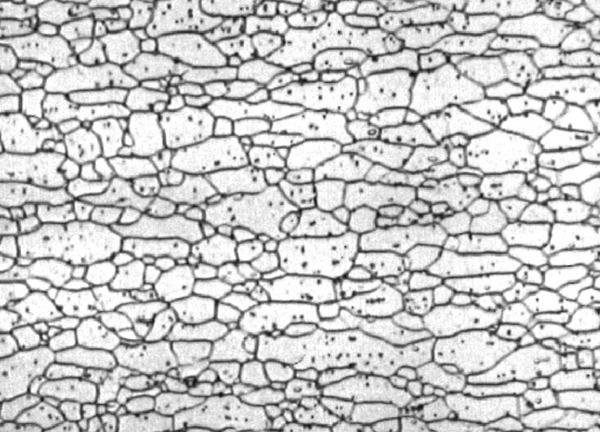
The microstructure of an HSLA340 cold rolled steel is available here. Download it and put it into your current work directory.
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import cm
from scipy import ndimage
import pandas as pd
import os
%matplotlib nbagg
name = "HSLA 340.jpg"
workdir = "./"
files = os.listdir(workdir)
if name in files:
print("Ok, the file is in {0}".format(files))
else:
print("The file is not in {0} , retry !".format(files))
Ok, the file is in ['coins.jpg', '_data', 'HSLA 340.jpg', '05_Image_Processing_Tutorial.ipynb', '.ipynb_checkpoints', '00_Basics.ipynb', '04_Examples', 'dices.tif', '_tests', '08_Image_Processing_Practical_Work.ipynb', '02_Advanced_Examples']
im = np.array(Image.open(workdir + name))
fig, ax = plt.subplots()
ax.axis("off")
plt.imshow(im, cmap = cm.copper)
plt.colorbar()
plt.show()
<IPython.core.display.Javascript object>
1) Histogram¶
Plot the histogram of the image and find the right level for the thresholding.
2) Thresholding¶
Use thresholding to convert the image to binary format.
3) Labeling¶
Use the ndimage.measurements.label
to label each grain and plot the
result.
4) Grain size measurement¶
Use the labeled image to measure the size (in pixels) of each grain. Create a new image on which the color of each pixel indicates the size of the grain it belongs to.
5) Grain shape factor and orientation¶
The cold rolling treatment modifies the shape factor as well as their orientation. Find a way to measure and represent the shape factor of each grain and to mesure their orientation.